The HTML5 Web Sockets represent the next evolution of web communications. A WebSocket is a full duplex, bidirectional communication channel that operates over a single TCP socket. It has become a standard for building real time web applications. In ColdFusion 10, a messaging layer has been provided that implements the Web Socket protocol. It enables you to build applications that are based on publisher/subscriber model and applications that are based on subscriber model wherein a push from a server is possible.
Publisher/Subscriber model
In this broadcast based method, publisher posts a message and a subscriber consumes it. A subscriber subscribes to a channel to which a publisher posts the message. A subscriber can subscribe to multiple channels and consume the data sent through the channel:
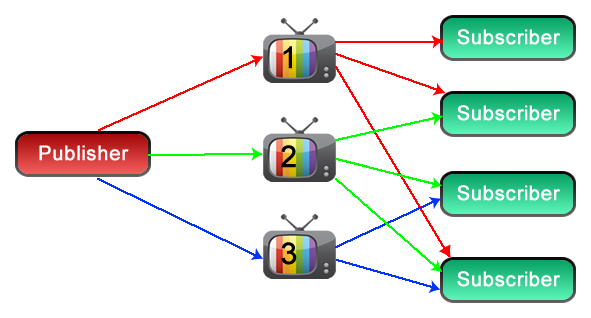
To implement the broadcast based approach in ColdFusion, you can specify the channel details in Application.cfc file, use the new tag - cfwebsocket to create a websocket and then send, receive and manage the connection through various JavaScript functions.
Application.cfc:
The websocket channels through which the communication takes place between the publisher and subscriber is specified in the wschannels variable. As observed in the above code snippet, the channel names are specified in a struct. Optionally, you can also specify the cfclistener key which contains channel listener functions for each channel (I plan to cover this workflow in my next post).
Creating a WebSocket:
To create a websocket, the cfwebsocket tag is used:
The cfwebsocket tag will establish a connection with the WebSocket Server and the server will respond with a ‘Welcome’ message; acknowledging the publisher or subscriber that the connection has been established. As observed in the above code snippet various handlers are specified. These are JavaScript functions that the user has to implement. The onMessage handler (messageHandler in code) is called when the server responds with a message or when a data is received through the subscribed channel. The complete workflow is as shown below:
JavaScript Functions:
Once a connection is established with the server, the websocket object is then used to manage the connection. Here is list of JavaScript functions (please note this is not a complete list, only those relevant to the example are mentioned):
openConnection
By default, a connection is established with the server when the cfwebsocket tag is specified in the page. If the user chooses to close the connection (see closeConnection function below) and then establish again, then this function should be called on the socket object. Example:
socket.openConnection();
isConnectionOpen
This function is used to check whether the connection is established with the server. It returns a boolean value true if the connection is established or false if the connection is closed. Example:
socket.closeConnection();
subscribe
Instead of specifying the channels to subscribe in the subscribeTo attribute of the cfwebsocket tag, you can use the JavaScript function ‘subscribe’ to subscribe to a channel. This function optionally takes two arguments – subscriberInfo and messageHandler. Subscriber information can include subscriber related data such as id,name,age in a struct. If a messageHandler function is specified then whenever a message is received through the channel, the messageHandler would be invoked. Example:
socket.subscribe(“channel1”,{name: ‘Sagar’, id: ‘888’}, channelHandler);
unsubscribe
The ‘unsubscribe’ function is used to unsubscribe from a channel. Once a user unsubscribes from the channel, the messages from the channel are no longer delivered to the client. Example:
socket.unsubscribe(“channel1”,{name: ‘Sagar’, id: ‘888’});
publish
The publisher can send data through a channel using the ‘publish’ method. The Server acknowledges this action by sending an acknowledgement to the publisher. The third argument of the publish method is a struct containing the publisher information. Again this is an optional argument. Example:
socket.publish(“channel1”,”message_here”,{name: ‘Publisher’, id: ‘222’});
closeConnection
The user can close the connection by calling the ‘closeConnection’ function on the socket object. This function will close the connection with the server and the user will not receive any messages. If a user wants to open the connection then the ‘openConnection’ function should be called. Example:
socket.closeConnection();
I have created a sample application, you can download it here. Open two browser instances and run the cfm file. You’ll be able to see the communication taking place between the two instances when some message is sent through the channel.
Publisher/Subscriber model
In this broadcast based method, publisher posts a message and a subscriber consumes it. A subscriber subscribes to a channel to which a publisher posts the message. A subscriber can subscribe to multiple channels and consume the data sent through the channel:
To implement the broadcast based approach in ColdFusion, you can specify the channel details in Application.cfc file, use the new tag - cfwebsocket to create a websocket and then send, receive and manage the connection through various JavaScript functions.
Application.cfc:
component {
this.name = "WebSocketAPP";
this.wschannels = [{name="channel1"},{name="channel2"},{name="channel3"}]; }
this.name = "WebSocketAPP";
this.wschannels = [{name="channel1"},{name="channel2"},{name="channel3"}]; }
The websocket channels through which the communication takes place between the publisher and subscriber is specified in the wschannels variable. As observed in the above code snippet, the channel names are specified in a struct. Optionally, you can also specify the cfclistener key which contains channel listener functions for each channel (I plan to cover this workflow in my next post).
Creating a WebSocket:
To create a websocket, the cfwebsocket tag is used:
<cfwebsocket name="socket"
onMessage="messageHandler"
onOpen="openHandler"
onClose="closeHandler"
onError="errorHandler"
subscribeTo="channel1,channel2">
The cfwebsocket tag will establish a connection with the WebSocket Server and the server will respond with a ‘Welcome’ message; acknowledging the publisher or subscriber that the connection has been established. As observed in the above code snippet various handlers are specified. These are JavaScript functions that the user has to implement. The onMessage handler (messageHandler in code) is called when the server responds with a message or when a data is received through the subscribed channel. The complete workflow is as shown below:
JavaScript Functions:
Once a connection is established with the server, the websocket object is then used to manage the connection. Here is list of JavaScript functions (please note this is not a complete list, only those relevant to the example are mentioned):
openConnection
By default, a connection is established with the server when the cfwebsocket tag is specified in the page. If the user chooses to close the connection (see closeConnection function below) and then establish again, then this function should be called on the socket object. Example:
socket.openConnection();
isConnectionOpen
This function is used to check whether the connection is established with the server. It returns a boolean value true if the connection is established or false if the connection is closed. Example:
socket.closeConnection();
subscribe
Instead of specifying the channels to subscribe in the subscribeTo attribute of the cfwebsocket tag, you can use the JavaScript function ‘subscribe’ to subscribe to a channel. This function optionally takes two arguments – subscriberInfo and messageHandler. Subscriber information can include subscriber related data such as id,name,age in a struct. If a messageHandler function is specified then whenever a message is received through the channel, the messageHandler would be invoked. Example:
socket.subscribe(“channel1”,{name: ‘Sagar’, id: ‘888’}, channelHandler);
unsubscribe
The ‘unsubscribe’ function is used to unsubscribe from a channel. Once a user unsubscribes from the channel, the messages from the channel are no longer delivered to the client. Example:
socket.unsubscribe(“channel1”,{name: ‘Sagar’, id: ‘888’});
publish
The publisher can send data through a channel using the ‘publish’ method. The Server acknowledges this action by sending an acknowledgement to the publisher. The third argument of the publish method is a struct containing the publisher information. Again this is an optional argument. Example:
socket.publish(“channel1”,”message_here”,{name: ‘Publisher’, id: ‘222’});
closeConnection
The user can close the connection by calling the ‘closeConnection’ function on the socket object. This function will close the connection with the server and the user will not receive any messages. If a user wants to open the connection then the ‘openConnection’ function should be called. Example:
socket.closeConnection();
Working Example
I have created a sample application, you can download it here. Open two browser instances and run the cfm file. You’ll be able to see the communication taking place between the two instances when some message is sent through the channel.
Great post - it's starting to make sense. When you pass optional data with the Subscribe(), do you *also* have to pass that data along to the Unsubscribe() for the channel to be unsubscribed?
ReplyDeleteBen, the subscriber info provided in subscribe and unsubscribe methods come handy when dealing with user defined Channel listeners. A channel listener is a CFC containing methods which are called when a WebSocket object's JavaScript functions are called. The methods in CFC can take the decision (to allow subscribing) based on the info included in the subscribe function.
ReplyDeleteFor the ones without user defined channel listeners it is not required to provide subscriber info.
Ok cool - I'm gonna be working on some WS stuff today, see if I can wrap my head around it.
ReplyDeleteIs there websockets support in CF 9
ReplyDeleteNo. It was added in ColdFusion 10.
ReplyDeleteWhat is the limitations to the number of channels we can have. Also can we have dynamic channels like ch.id1, ch.id2 ....ch.idn
ReplyDeletethe number of channels being dependent on the number of user groups that are logged in from id1 to idn. What is the limitation to the number of channels. Each group ch.id1 etc. could have any number of subscribers 1 to 10 say.